Before going to programming , we need to understand the events used in mouse
1.mouseListener
mouseClicked
mouseEntered
mouseExited
mousePressed
mouseReleased
2.mouseMotionListener
mouseDragged(MouseEvent event)
mouseMoved(MouseEvent event)
For more information goto
http://stackoverflow.com/questions/1798582/difference-between-mouselistener-and-mousemotionlistener-in-java
If we are using mouseListener or mouseMotionListener, then we need to implements all its abstract methodes
If we have only needed one method (, for eg:-mouseClicked ) then also we must use all abstract methods in programming otherwise we can use actionListener. we can use actionListener in next tutorial
so that you can understand the events very well
First create a class EventDemo
→just right click on package name "javacourse"→New→Java Class→Name: EventDemo→Click finish
Now you can see a yellow bulb with red ball on left side→click on it
then you can see
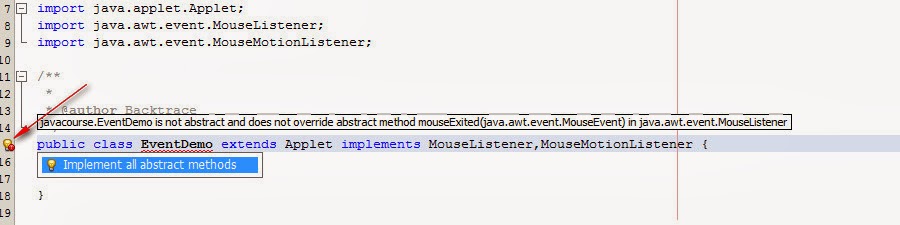
After you click that "implements all abstract methods"
you get like this code
1.mouseListener
mouseClicked
mouseEntered
mouseExited
mousePressed
mouseReleased
2.mouseMotionListener
mouseDragged(MouseEvent event)
mouseMoved(MouseEvent event)
For more information goto
http://stackoverflow.com/questions/1798582/difference-between-mouselistener-and-mousemotionlistener-in-java
If we are using mouseListener or mouseMotionListener, then we need to implements all its abstract methodes
If we have only needed one method (, for eg:-mouseClicked ) then also we must use all abstract methods in programming otherwise we can use actionListener. we can use actionListener in next tutorial
so that you can understand the events very well
First create a class EventDemo
→just right click on package name "javacourse"→New→Java Class→Name: EventDemo→Click finish
Now you can see a yellow bulb with red ball on left side→click on it
then you can see
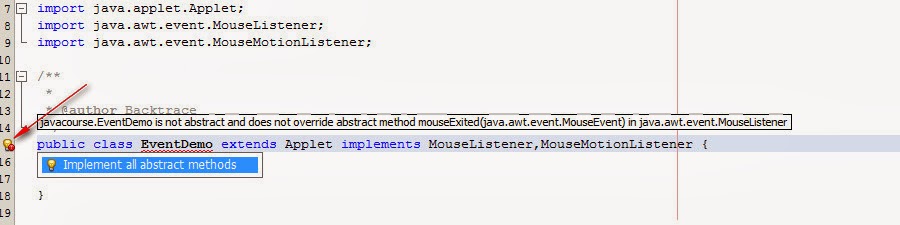
After you click that "implements all abstract methods"
you get like this code
import java.applet.Applet; import java.awt.event.MouseEvent; import java.awt.event.MouseListener; import java.awt.event.MouseMotionListener; /** * * @author Backtrace */ public class EventDemo extends Applet implements MouseListener,MouseMotionListener { @Override public void mouseClicked(MouseEvent e) { throw new UnsupportedOperationException("Not supported yet."); } @Override public void mousePressed(MouseEvent e) { throw new UnsupportedOperationException("Not supported yet."); } @Override public void mouseReleased(MouseEvent e) { throw new UnsupportedOperationException("Not supported yet."); } @Override public void mouseEntered(MouseEvent e) { throw new UnsupportedOperationException("Not supported yet."); } @Override public void mouseExited(MouseEvent e) { throw new UnsupportedOperationException("Not supported yet."); } @Override public void mouseDragged(MouseEvent e) { throw new UnsupportedOperationException("Not supported yet."); } @Override public void mouseMoved(MouseEvent e) { throw new UnsupportedOperationException("Not supported yet."); } }
Now we can use this function to show what you have done with mouse
showStatus("mouse clicked"+e.getX()+"."+e.getY());
So your code can change like this
import java.applet.Applet; import java.awt.event.*; import java.awt.event.MouseEvent; import java.awt.event.MouseListener; import java.awt.event.MouseMotionListener; /** * * @author Backtrace */ public class EventDemo extends Applet implements MouseListener,MouseMotionListener { public void init(){ addMouseListener(this); addMouseMotionListener(this); } @Override public void mouseClicked(MouseEvent e) { showStatus("mouse clicked "+e.getX()+"."+e.getY()); } @Override public void mousePressed(MouseEvent e) { showStatus("mouse pressed "+e.getX()+"."+e.getY()); } @Override public void mouseReleased(MouseEvent e) { showStatus("mouse release "+e.getX()+"."+e.getY()); } @Override public void mouseEntered(MouseEvent e) { showStatus("mouse entered "+e.getX()+"."+e.getY()); } @Override public void mouseExited(MouseEvent e) { showStatus("mouse Exited "+e.getX()+"."+e.getY()); } @Override public void mouseDragged(MouseEvent e) { showStatus("mouse dragged "+e.getX()+"."+e.getY()); } @Override public void mouseMoved(MouseEvent e) { showStatus("mouse moved "+e.getX()+"."+e.getY()); } }
Output
----------------
0 Comments:
Post a Comment